How To Write Dynamic XPath In Selenium WebDriver
It’s important for automated testing to be able to identify the web elements of an Application Under Test (AUT). It may take a long time and experience to learn how to find web elements manually. Here we will show you how to find web elements using XPath in Selenium manually and easily.+
One of the useful functionalities in Selenium is locators. Locators allow us to find web elements. If the web elements are not found by the locators such as id, classname, name, link text etc., then we use XPath to find web elements on the web page.
Before learning how to write dynamic XPath in Selenium automation, we will learn what is XPath locator in Selenium and the following
What is XPath in Selenium?
XPath is designed to allow the navigation of XML documents, with the purpose of selecting individual elements, attributes, or some other part of an XML document for specific processing. XPath produces reliable locators but in performance wise it is slower (especially in IE older versions) compared to CSS Selector.
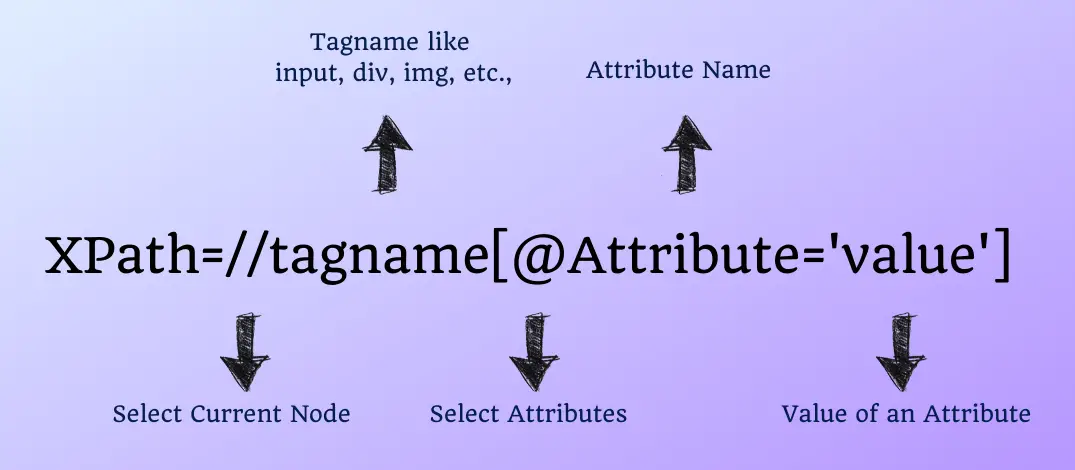
Syntax for XPath Selenium:
Xpath=//tagname[@Attribute='value']
- // – Select current node.
- Tagname – Tagname of an appropriate node.
- @ – Select attribute.
- Attribute – Attribute name of the node.
- Value – Value of the attribute.
Syntax:
findElement(By.xpath("XPath"));
Don’t miss Chrome Extensions To Find XPath
What are the Types of XPath?
There are two types of XPath in Selenium
- Absolute XPath
- Relative XPath
Note: Interviewers may ask you the difference between Absolute XPath & Relative XPath.
#1. Absolute XPath
Absolute XPath starts from the root node and ends with desired descendant element’s node. It starts with the top HTML node and ends with the input node. It starts with a single forward-slash(/) as shown below.
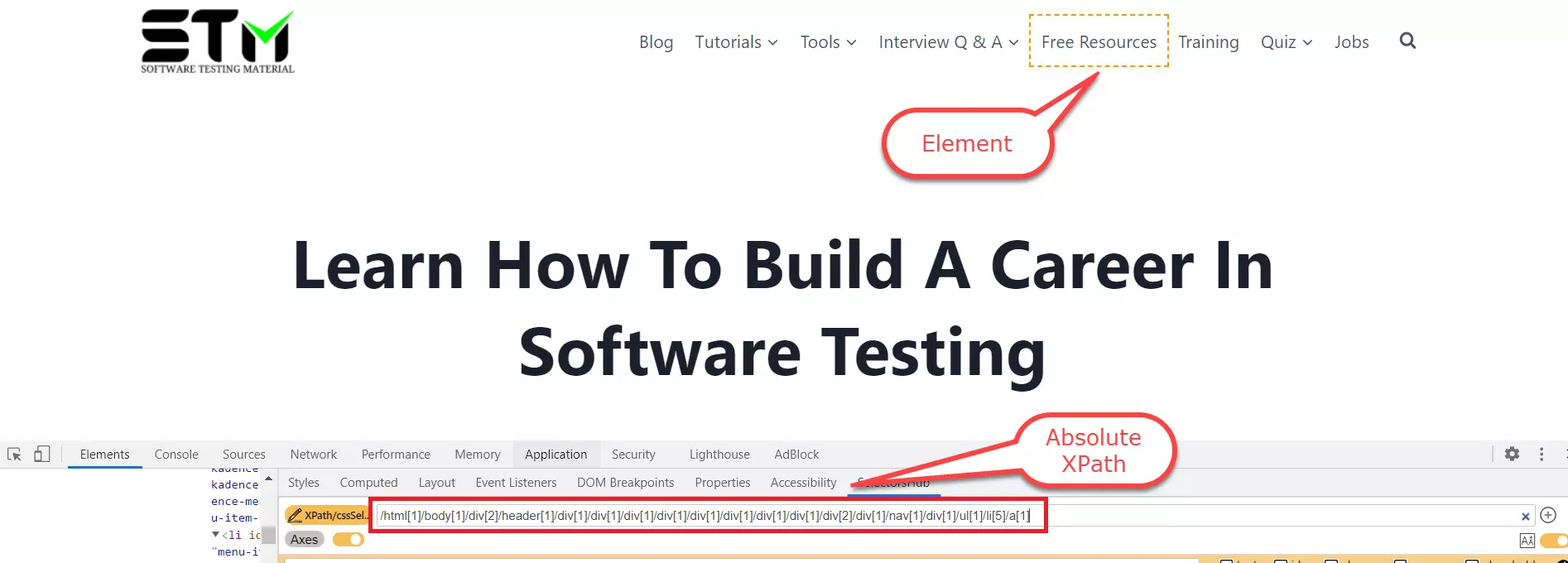
Absolute XPath Example
/html[1]/body[1]/div[2]/header[1]/div[1]/div[1]/div[1]/div[1]/div[1]/div[1]/div[1]/div[1]/div[2]/div[1]/nav[1]/div[1]/ul[1]/li[5]/a[1]
Another example
/html/body/div/div/form/table/tbody/tr/td/input
#2. Relative XPath
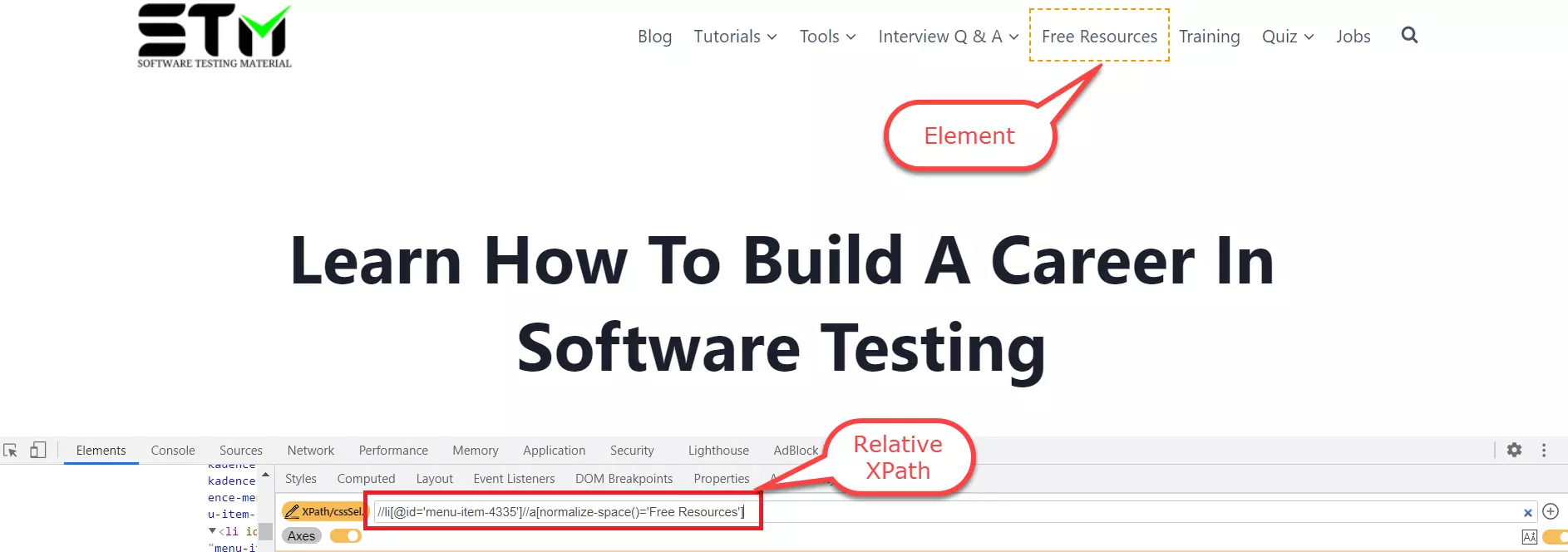
Relative XPath starts from any node in between the HTML page to the current element’s node(last node of the element). It starts with a double forward-slash(//) as shown below.
Relative XPath Example
//li[@id='menu-item-4335']//a[normalize-space()='Free Resources']
Another example
//input@id='email']
What is Dynamic XPath in Selenium & How to Find XPath?
Sometimes, we may not identify the element using the locators such as id, class, name, etc. In those cases, we use XPath to find an element on the web page.
At times, XPath may change dynamically and we need to handle the elements while writing scripts.
The standard way of writing XPath may not work and we need to write dynamic XPath in selenium scripts.
What are XPath axes?
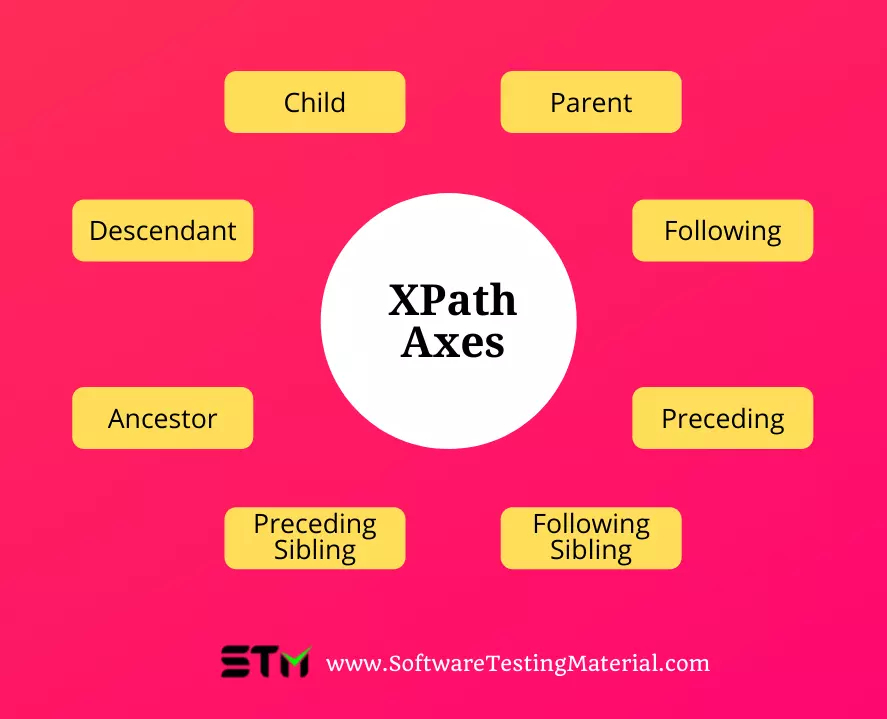
The XPath axes search different nodes in the XML document from the context (current) node. It is utilized to locate the node that is relative to the node on that tree.
XPath axes are the methods used to find dynamic elements that would otherwise be impossible using standard XPath methods, which do not include an ID, Classname, Name, or other identifiers.
Axes methods are used to find those elements, which dynamically change on refresh or any other operations. There are few axes methods commonly used in Selenium Webdriver like child, parent, ancestor, sibling, preceding, self, etc.
One of the challenging and time-consuming tasks in test automation is to modify test scripts when the AUT is changed, especially in the early stages of software development. Developers may change identifiers and elements quite often from one build to another. In addition, during the execution, the AUT’s elements may change dynamically.
To deal with these challenges, automation testers should not set fixed XPaths for elements in test cases, but instead, scripting XPaths dynamically based on certain patterns.
Axis Name | Description |
---|---|
ancestor | Shows all the ancestors (parent, grandparent, etc.,) related to the context (current) node. |
ancestor-or-self | Shows the context (current) node and all the ancestors. |
attribute | Shows all the atributes of the context (current) node. |
child | Shows all the children of the context (current) node. |
descendant | Specifies all descendants (children, grandchildren, etc.) of the context (current) node. |
descendant-or-self | Specifies all descendants (children, grandchildren, etc.) of the context (current) node and the current node itself. |
following | Specifies all the nodes that appear after the context (current) node. |
following-sibling | Specifies all siblings after the context (current) node. |
namespace | Specifies all the namespace nodes of the context (current) node. |
parent | Specifies the parent of the context (current) node. |
preceding | Specifies all the nodes that appear before the context (current) node in the HTML DOM structure. This does not specify ancestor, attribute, and namespace. |
preceding-sibling | Specifies all the sibling nodes that appear before the context (current) node in the HTML DOM structure. This does not specify descendent, attribute and namespace. |
self | Specifies the context (current) node. |
Different ways of writing Dynamic XPath in Selenium with examples
Let’s see the different ways of writing dynamic XPath in Selenium with examples:
- Using Single Slash
- Using Double Slash
- Using Single Attribute
- Using Multiple Attribute
- Using AND
- Using OR
- Using contains()
- Using starts-with()
- Using text()
- Using last()
- Using position()
- Using index
- Using following XPath axes
- Using preceding XPath axes
Learn How To Write Dynamic CSS Selector In Selenium WebDriver [Without Any Tool]
Here is a video tutorial to learn “Creating dynamic XPath in Selenium WebDriver”:
If you liked this video, then please subscribe to our YouTube Channel for more video tutorials.
Here I am trying to find the element (‘Email or Phone’ field) on Gmail Login Page
HTML Code: (Gmail login page – Email field)
<input id="Email" type="email" value="" spellcheck="false" class="emailClass"
autofocus="" name="Email" placeholder="Enter your email"/>
#1. Using Single Slash
This mechanism is also known as finding elements using Absolute XPath.
Single slash is used to create XPath with absolute path i.e. the XPath would be created to start selection from the document node/start node/parent node.
Syntax:
html/body/div[1]/div[2]/div[2]/div[1]/form/div[1]/div/div[1]/div/div/input[1]
#2. Using Double Slash
This mechanism is also known as finding elements using Relative XPath.
Double slash is used to create XPath with relative path i.e. the XPath would be created to start selection from anywhere within the document. – Search in a whole page (DOM) for the preceding string
Syntax:
//form/div[1]/div/div[1]/div/div/input[1]
#3. Using Single Attribute
You could write the syntax in two ways as mentioned below. Including or excluding HTML Tag. If you want to exclude HTML Tag then you need to use *
Syntax:
//<HTML tag>[@attribute_name='attribute_value']
or
//*[@attribute_name='attribute_value']
Note: ‘*‘ after double slash is to match any tag with the desired text
XPath based on above HTML:
//input[@id='Email']
or
//*[@id='Email']
#4. Using Multiple Attribute
Syntax:
//<HTML tag>[@attribute_name1='attribute_value1'][@attribute_name2='attribute_value2]
or
//*[@attribute_name1='attribute_value1'][@attribute_name2='attribute_value2]
XPath based on above HTML:
//input[@id='Email'][@name='Email']
or
//*[@id='Email'][@name='Email']
#5. Using AND
Syntax:
//<HTML tag>[@attribute_name1='attribute_value1' and @attribute_name2='attribute_value2] or //*[@attribute_name1='attribute_value1' and @attribute_name2='attribute_value2]
XPath based on above HTML:
//input[@id='Email' and @name='Email']
or
//*[@id='Email' and @name='Email']
#6. Using OR
Syntax:
//<HTML tag>[@attribute_name1='attribute_value1' or @attribute_name2='attribute_value2] or //*[@attribute_name1='attribute_value1' or @attribute_name2='attribute_value2]
XPath based on above HTML:
//input[@id='Email' or @name='Email']
or
//*[@id='Email' or @name='Email']
#7. Using contains()
Contains() is a method used to identify an element that changes dynamically and when we are familiar with some part of the attribute value of that element.
Syntax:
//<HTML tag>[contains(@attribute_name,'attribute_value')]
or
//*[contains(@attribute_name,'attribute_value')]
XPath based on above HTML:
//input[contains(@id,'Email')]
or
//*[contains(@id,'Email')]
or
//input[contains(@name,'Email')]
or
//*input[contains(@name,'Email')]
#8. Using starts-with()
starts-with() method is used to identify an element when we are familiar with the value of the attribute (starting with the specified text) of an element.
Syntax:
//<HTML tag>[starts-with(@attribute_name,'attribute_value')]
or
//*[starts-with(@attribute_name,'attribute_value')]
XPath based on above HTML:
//input[starts-with(@id,'Ema')]
or
//*[starts-with(@id,'Ema')]
#9. Using text()
This mechanism is used to locate an element based on the text available on a webpage
As per the above image, we could identify the elements text based on the below xpath.
//*[text()='New look for sign-in coming soon']
#10. Using last()
Selects the last element (of mentioned type) out of all input element present
To identify the element (last text field ) “Your current email address”, we could use the below xpath.
findElement(By.xpath("(//input[@type='text'])[last()]"))
To identify the element “Year”, we could use the below xpath.
[last()-1] – Selects the last but one element (of mentioned type) out of all input element present
findElement(By.xpath("(//input[@type='text'])[last()-1]"))
#11. Using position()
Selects the element out of all input element present depending on the position number provided
In below given xpath, [@type=’text’] will locate text field and function [position()=2] will locate text filed which is located on 2nd position from the top.
findElement(By.xpath("(//input[@type='text'])[position()=2]"))
or
findElement(By.xpath("(//input[@type='text'])[2]"))
#12. Using index
By providing the index position in the square brackets, we could move to the nth element. Based on the below xpath, we could identify the Last Name field.
findElement(By.xpath("//label[2]"))
#13. Using following XPath axes
By using this we could select everything on the web page after the closing tag of the current node
xpath of the FirstName field is as follows
//*[@id='FirstName']
To identify the input field of type text after the FirstName field, we need to use the below xpath.
//*[@id='FirstName']/following::input[@type='text']
Here I used, following xpath axes and two colons and then specified the required tag (i.e., input)
To identify just the input field after the FirstName field, we need to use the below xpath.
//*[@id='FirstName']/following::input
#14. Using preceding XPath axes
Selects all nodes that appear before the current node in the document, except ancestors, attribute nodes, and namespace nodes
xpath of the LastName field is as follows
//*[@id='LastName']
To identify the input field of type text before the LastName field, we need to use the below xpath.
//*[@id='LastName']//preceding::input[@type='text']"
Here I used, preceding xpath axes and two colons and then specified the required tag (i.e., input).
Conclusion
XPath in Selenium is used to locate the elements when other locators wont work.
Two types of Selenium XPath are
- 1. Absolute XPath
- 2. Relative XPath.
The different ways of finding the elements on a webpage using the Selenium XPath discussed here will be useful when you work in your real-time project.
If you have any queries, please comment below in the comment section. Like this post? Don’t forget to share it!
Here are few hand-picked articles for you to read next:
Selenium Tutorial
TestNG Tutorial
Selenium Interview Questions
TestNG Interview Questions
Manual Testing Tutorial
Manual Testing Interview Questions
Superb!!! Thanks for sharing this great information.
Glad you liked it Sreenivas. Keep visiting.
Great Post!!!!!! You saved my times , do include some demos of xpath axes
Thanks Rahul. I am glad you found it helpful. Yeah, we have a plan to write an article on xpath axes. Subscribe our blog for latest updates.
Thanks Raj Kumar
Excellent video and info is good
Thanks for your kind words Prasad
Thanks Raj,
It helps me alot.
Great job.
I am glad it helped. Keep visiting our site Santhosh.
helpful post. saved my time
thank you Rajkumar.
In 5th) Using AND:
@attribute_name2=’attribute_value2] ===> @attribute_name2=’attribute_value2′]
Ending single quote (‘) is missing.
Thanks will update it
Very nice explaination about xpath.
Its really very much helpfull for us.
thanks a lot .
Will it will be helpful for finding xpath for any dynamic text or link with the help of following and preceding xpath which are not dynamic in nature ?
Yes