Python Join() Method with Examples
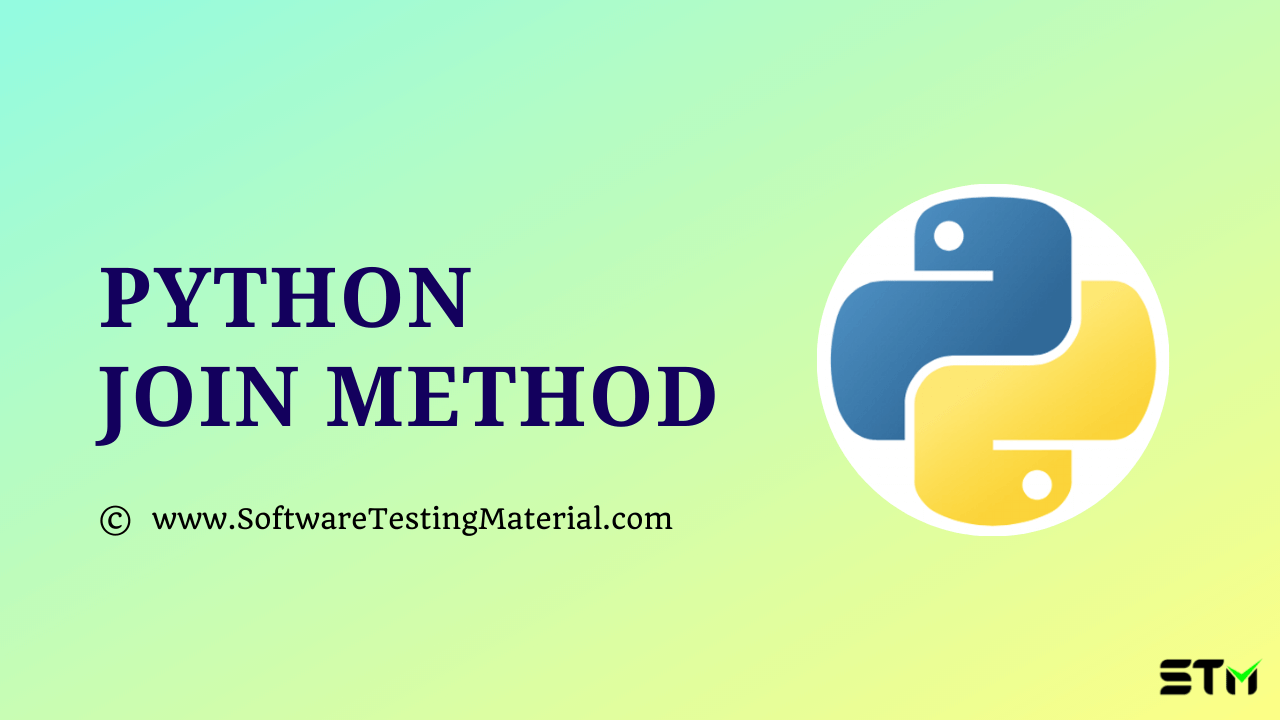
In this article, we will see what is Python Join method with examples. This Python join() method tutorial helps you in implementing this concept and get a tight grip over this.
Python String join() Method
It allows an iterable as the input parameter and joins their elements into a string with some of the string separator such as comma, space, underscores, etc., We can also send a string value such as “ABC” or “XYZ” etc., It returns a string by concatenating all the elements of an iterable, separated by a string separator. It joins a sequence of types like List, Tuple, String, Dictionary, & Set and converts them into a String.
Join() Method Syntax:
Following is the syntax of join() method.
str.join(iterable)
String: str is the name of the string in which joined elements of iterable will be stored.
Parameter: Iterable is a parameter and we have to pass only iterable parameter. The returned values are strings. Some examples are List, Tuple, String, Dictionary, and Set.
Return Value: It returns a string by concatenating all the elements of an iterable, separated by a string separator.
Type Error: If the iterable contains any non-string values, it raises an exception called TypeError exception.
Python string method join() with Examples
Let’s move forward and see some examples using join method by taking a sequence of each data type one by one.
Example 1: The join() method with List as Iterable
# .join() with lists list = ['1', '2', '3', '4'] separator = ', ' print(separator.join(list))
Output:
1, 2, 3, 4
Example 2: The join() method with Tuple as Iterable
# .join() with tuple tuple = ("Software", "Testing", "Material") seperator = '_' output = seperator.join(tuple) print(output)
Output:
Software_Testing_Material
Example 3: The join() method with dictionaries as Iterable
The join() can take a dict and concatenate all its key fields as one.
# .join() with dictionaries inputDict = {'software': 1, 'testing': 2} separator = '_' Output = separator.join(inputDict) print("After join: " + Output) print("Join() return type: {}".format(type(Output)))
Output:
After join: software_testing Join() return type: <class 'str'>
Example 4: The join() method with Set as Iterable
# .join() with sets inputSet1 = {'2', '1', '3'} separtor1 = ', ' print(separtor1.join(inputSet1)) inputSet2 = {'Java', 'Python', 'Ruby'} separtor2 = '-' print(separtor2.join(inputSet2))
Output:
1, 3, 2 Python-Java-Ruby
Also Read:
- Python Strings
- Python Data Types
- Python Multiline String
- Python Interview Questions
- How to Convert Python List to String (4 Ways)
- Python float() Function Explained with Examples